
ePortefolio Ida Brandão
Arduino Programming
Tests
Arduino - Led Blink
After testing with Tinkercad Simulator I've connected my Arduino circuit board to my computer and opened the Arduino software and tested the first basic example with a LED blinking
Tinkercad test - Traffic Light Sequence
Arduino Traffic Light Sequence
Arduino traffic light cars and pedestrians
Examples similar to the one proposed in Week 2 -
https://www.14core.com/making-an-interactive-traffic-lights-using-arduino-with-code/
http://katak-tempurung.blogspot.com/2011/07/pedestrian-interactive-traffic-light.html
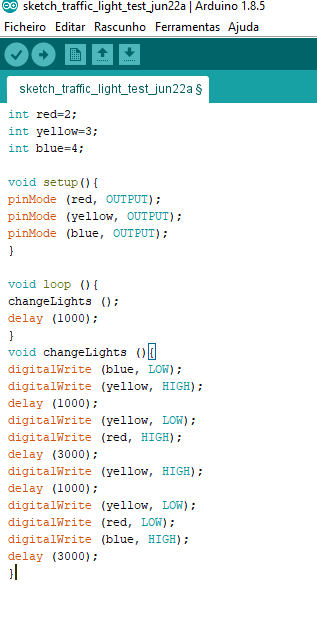
Code
int carRed = 12;
int carYellow = 11;
int carGreen = 10;
int pedRed = 9;
int pedGreen = 8;
int button = 2;
int crossTime = 5000;
unsigned long changeTime;
int x;
void setup(){
pinMode(carRed, OUTPUT);
pinMode(carYellow, OUTPUT);
pinMode(carGreen, OUTPUT);
pinMode(pedRed, OUTPUT);
pinMode(pedGreen, OUTPUT);
pinMode(button, INPUT);
digitalWrite(carGreen, HIGH);
digitalWrite(pedRed, HIGH);
}
void loop(){
int state = digitalRead(button);
if (state == HIGH && (millis() - changeTime) > 5000){
changeLights();
}
}
void changeLights(){
digitalWrite(carGreen, LOW);
digitalWrite(carYellow, HIGH);
delay(2000);
digitalWrite(carYellow, LOW);
digitalWrite(carRed, HIGH);
delay(1000);
digitalWrite(pedRed, LOW);
digitalWrite(pedGreen, HIGH);
delay(crossTime);
for(int x=0; x<10; x++){
digitalWrite(pedGreen, HIGH);
delay(250);
digitalWrite(pedGreen, LOW);
delay(250);
}
digitalWrite(pedRed, HIGH);
delay(500);
digitalWrite(carYellow, HIGH);
digitalWrite(carRed, LOW);
delay(1000);
digitalWrite(carGreen, HIGH);
digitalWrite(carYellow, LOW);
changeTime = millis();
}
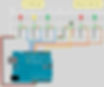
Test in Tinkercad simulator
Circuit test
Arduino - Photoresistor - LED brightness
Testing LED brightness with photoresistor in Tinkercad and Arduino IDE

Arduino alarm with LED-LDR-Buzzer

Arduino servomotor

Colour shifting obstacle detector using ultrasonic sensor - Arduino Hub
It is a simple project to detect obstacles in various distances. The sonar sensor detects the distance between the object and the board and based on the distance from the board it lights up Red, Yellow and Green LED.
When the object is too close to the board (10 centimeters) it lights up Red LED as an indication of caution.
It lights up Yellow LED when the object is between 11-100 centimeter. If the object is detected outside of the 100-centimeter range, it lights up Green LED as an indication of the object in safe range.
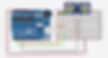
Materials
-
Arduino UNO (1)
-
HC-SR04 Ultrasonic Sensor (1)
-
Red LED (1)
-
Yellow LED (1)
-
Green LED (1)
-
220 Ohm Resistors (3)
-
Breadboard (1)
-
Male to Male Hookup wires (10)
-
A Ruler that measures centimeters
CODE
int green=2,yellow=3,red=4, echo=5, trig=6, duration, distance;
int call_distance(){
digitalWrite(trig, LOW);
delayMicroseconds(2);
digitalWrite(trig, HIGH);
delayMicroseconds(10);
digitalWrite(trig, LOW);
duration=pulseIn(echo,HIGH);
distance=(duration/2)*.0344;
return distance;
}
void fade(int pin){
for(int f=0; f<=255; f+=30){
analogWrite(pin, f);
delay(30);
}
for(int f=255; f>0; f-=30){
analogWrite(pin, f);
delay(30);
}
}
void setup(){
Serial.begin(9600);
pinMode(green, OUTPUT);
pinMode(yellow, OUTPUT);
pinMode(red, OUTPUT);
pinMode(echo, INPUT);
pinMode(trig, OUTPUT);
}
void loop(){
call_distance();
while(distance<=10){
digitalWrite(yellow, LOW);
digitalWrite(green, LOW);
fade(red);
call_distance();
}
while(distance>10&&distance<=100){
digitalWrite(red, LOW);
digitalWrite(green, LOW);
fade(yellow);
call_distance();
}
while(distance>100){
digitalWrite(red, LOW);
digitalWrite(yellow, LOW);
fade(green);
call_distance();
}
delay(100);
}
Controlling multiple servo motors with Arduino
https://circuitdigest.com/microcontroller-projects/controlling-multiple-servo-motors-with-arduino
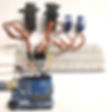
#include <Servo.h>
Servo servo1;
Servo servo2;
Servo servo3;
Servo servo4;
int i = 0;
void setup() {
servo1.attach(3);
servo2.attach(5);
servo3.attach(6);
servo4.attach(9);
}
void loop() {
for (i = 0; i < 180; i++) {
servo1.write(i);
servo2.write(i);
servo3.write(i);
servo4.write(i);
delay(10);
}
for (i = 180; i > 0; i--) {
servo1.write(i);
servo2.write(i);
servo3.write(i);
servo4.write(i);
delay(10);
}
}